Silhouette
Creating a node graph widget in Silhouette FX.
Note
Requires Silhouette 7.x
or above as previous versions don’t
come built-in with its own PySide2 build or provide access to the
QMainWindow
object.
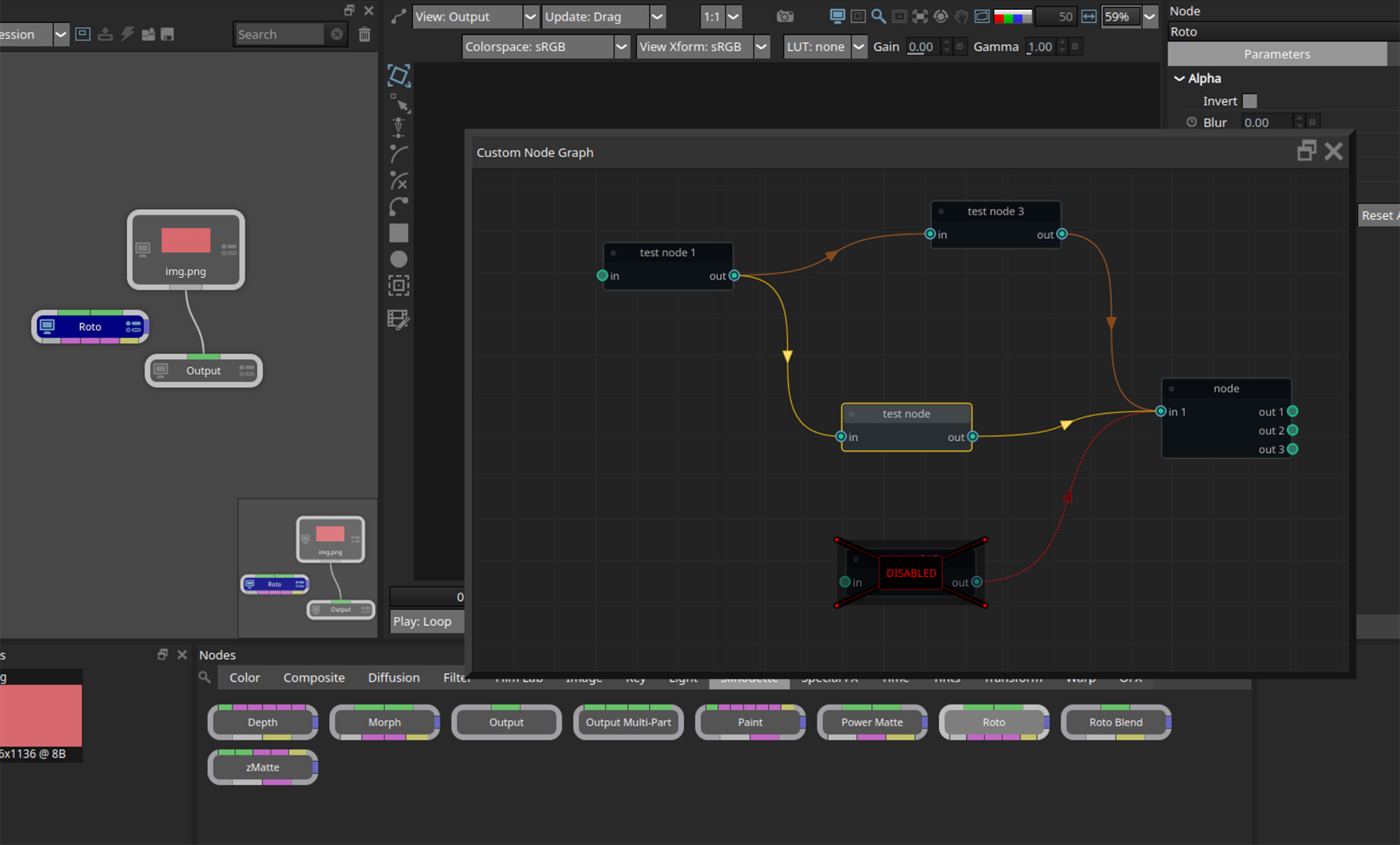
Here is an example where the
NodeGraphQt.NodeGraph.widget
can be
registered as a dockable panel in the application . 1import fx
2
3from Qt import QtWidgets, QtCore
4from NodeGraphQt import NodeGraph, BaseNode
5
6
7# create the widget wrapper that can be docked to the main window.
8class NodeGraphPanel(QtWidgets.QDockWidget):
9 """
10 Widget wrapper for the node graph that can be docked to
11 the main window.
12 """
13
14 def __init__(self, graph, parent=None):
15 super(NodeGraphPanel, self).__init__(parent)
16 self.setObjectName('nodeGraphQt.NodeGraphPanel')
17 self.setWindowTitle('Custom Node Graph')
18 self.setWidget(graph.widget)
19
20
21# create a simple test node class.
22class TestNode(BaseNode):
23
24 __identifier__ = 'nodes.silhouettefx'
25 NODE_NAME = 'test node'
26
27 def __init__(self):
28 super(TestNode, self).__init__()
29 self.add_input('in')
30 self.add_output('out')
31
32
33# create the node graph controller and register our "TestNode"
34graph = NodeGraph()
35graph.register_node(TestNode)
36
37# create nodes.
38node_1 = graph.create_node('nodes.silhouette.TestNode')
39node_2 = graph.create_node('nodes.silhouette.TestNode')
40node_3 = graph.create_node('nodes.silhouette.TestNode')
41
42# create the node graph panel that can be docked.
43sfx_graph_panel = NodeGraphPanel(graph)
44
45# add the doc widget into the main silhouette window.
46sfx_window = fx.ui.mainWindow()
47sfx_window.addDockWidget(QtCore.Qt.RightDockWidgetArea, sfx_graph_panel)