Menu Overview
NodeGraphQt
.Default Context Menu
The NodeGraphQt.NodeGraph
has a context menu can be accessed with
NodeGraph.context_menu()
.
It can also be populated it with a config file in JSON
format by using
NodeGraph.set_context_menu_from_file()
.
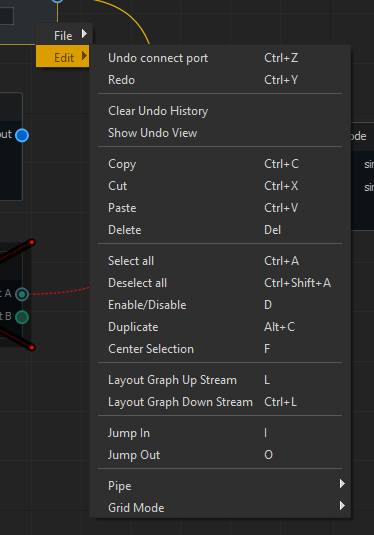
Adding to the Graph Menu
The "graph"
menu is the main context menu from the NodeGraph object, below
is an example where we add a "Foo"
menu and then a "Bar"
command with
the registered my_test()
function.
1from NodeGraphQt import NodeGraph
2
3# test function.
4def my_test(graph):
5 selected_nodes = graph.selected_nodes()
6 print('Number of nodes selected: {}'.format(len(selected_nodes)))
7
8# create node graph.
9node_graph = NodeGraph()
10
11# get the main context menu.
12context_menu = node_graph.get_context_menu('graph')
13
14# add a menu called "Foo".
15foo_menu = context_menu.add_menu('Foo')
16
17# add "Bar" command to the "Foo" menu.
18# we also assign a short cut key "Shift+t" for this example.
19foo_menu.add_command('Bar', my_test, 'Shift+t')
Adding to the Nodes Menu
Aside from the main context menu, the NodeGraph also has a nodes menu where you can override context menus on a per node type basis.
"io.github.jchanvfx.FooNode"
1from NodeGraphQt import BaseNode, NodeGraph, setup_context_menu
2
3# define a couple example nodes.
4class FooNode(BaseNode):
5
6 __identifier__ = 'io.github.jchanvfx'
7 NODE_NAME = 'foo node'
8
9 def __init__(self):
10 super(FooNode, self).__init__()
11 self.add_input('in')
12 self.add_output('out')
13
14class BarNode(FooNode):
15
16 NODE_NAME = 'bar node'
17
18# define a test function.
19def test_func(graph, node):
20 print('Clicked on node: {}'.format(node.name()))
21
22# create node graph and register node classes.
23node_graph = NodeGraph()
24node_graph.register_node(FooNode)
25node_graph.register_node(BarNode)
26
27# set up default menu commands.
28setup_context_menu(node_graph)
29
30# get the nodes menu.
31nodes_menu = node_graph.get_context_menu('nodes')
32
33# here we add override the context menu for "io.github.jchanvfx.FooNode".
34nodes_menu.add_command('Test',
35 func=test_func,
36 node_type='io.github.jchanvfx.FooNode')
37
38# create some nodes.
39foo_node = graph.create_node('io.github.jchanvfx.FooNode')
40bar_node = graph.create_node('io.github.jchanvfx', pos=[300, 100])
41
42# show widget.
43node_graph.widget.show()
Adding with Config files
Adding menus and commands can also be done through configs and python module files.
example python script containing a test function.
../path/to/my/hotkeys/cmd_functions.py
1def graph_command(graph):
2 """
3 function that's triggered on the node graph context menu.
4
5 Args:
6 graph (NodeGraphQt.NodeGraph): node graph controller.
7 """
8 print(graph)
9
10def node_command(graph, node):
11 """
12 function that's triggered on a node's node context menu.
13
14 Args:
15 graph (NodeGraphQt.NodeGraph): node graph controller.
16 node: (NodeGraphQt.NodeObject): node object triggered on.
17 """
18 print(graph)
19 print(node.name())
example json
config for the node graph context menu.
../path/to/my/hotkeys/graph_commands.json
1[
2 {
3 "type":"menu",
4 "label":"My Sub Menu",
5 "items":[
6 {
7 "type":"command",
8 "label":"Example Graph Command",
9 "file":"../examples/hotkeys/cmd_functions.py",
10 "function_name":"graph_command",
11 "shortcut":"Shift+t",
12 }
13 ]
14 }
15]
example json
config for the nodes context menu.
../path/to/my/hotkeys/node_commands.json
1[
2 {
3 "type":"command",
4 "label":"Example Graph Command",
5 "file":"../examples/hotkeys/cmd_functions.py",
6 "function_name":"node_command",
7 "node_type":"io.github.jchanvfx.FooNode",
8 }
9]
In the main code where your node graph controller is defined we can just call the
NodeGraph.set_context_menu_from_file()
1from NodeGraphQt import NodeGraph
2
3node_graph = NodeGraph()
4node_graph.set_context_menu_from_file(
5 '../path/to/a/hotkeys/graph_commands.json', menu='graph'
6)
7node_graph.set_context_menu_from_file(
8 '../path/to/a/hotkeys/node_commands.json', menu='nodes'
9)
Adding with Serialized data
Alternatively if you do not prefer to have json
config files the node graph also has a
NodeGraph.set_context_menu()
function.
here’s an example.
1from NodeGraphQt import NodeGraph
2
3data = [
4 {
5 'type': 'menu',
6 'label': 'My Sub Menu',
7 'items': [
8 {
9 'type': 'command',
10 'label': 'Example Graph Command',
11 'file': '../examples/hotkeys/cmd_functions.py',
12 'function_name': 'graph_command',
13 'shortcut': 'Shift+t'
14 },
15 ]
16 },
17]
18
19node_graph = NodeGraph()
20node_graph.set_context_menu(menu_name='graph', data)