Nuke
Creating a node graph widget in Nuke.
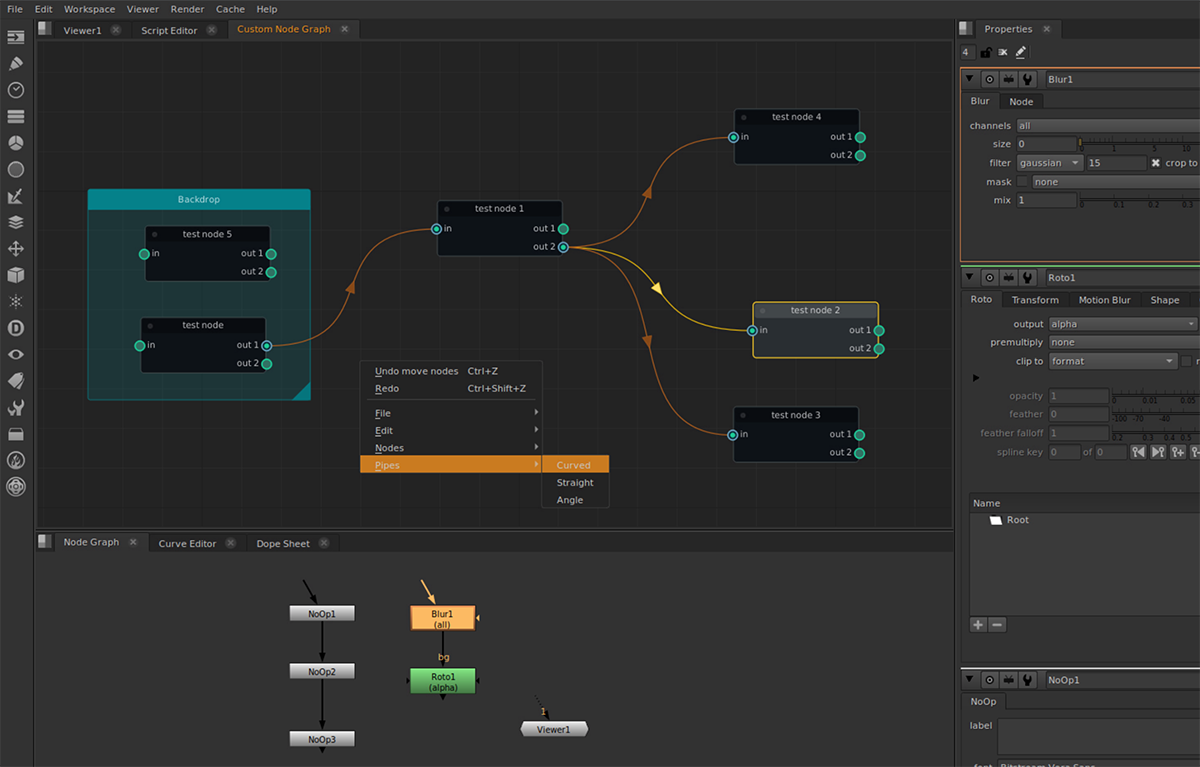
Here is an example where the
NodeGraphQt.NodeGraph.widget
can be
registered as a panel in the compositing application NUKE. 1from nukescripts import panels
2
3from Qt import QtWidgets, QtCore
4from NodeGraphQt import NodeGraph, BaseNode
5
6
7# create a simple test node class.
8class TestNode(BaseNode):
9
10 __identifier__ = 'nodes.nuke'
11 NODE_NAME = 'test node'
12
13 def __init__(self):
14 super(TestNode, self).__init__()
15 self.add_input('in')
16 self.add_output('out 1')
17 self.add_output('out 2')
18
19
20# create the node graph controller and register our "TestNode".
21graph = NodeGraph()
22graph.register_node(TestNode)
23
24# create nodes.
25node_1 = graph.create_node('nodes.nuke.TestNode')
26node_2 = graph.create_node('nodes.nuke.TestNode')
27node_3 = graph.create_node('nodes.nuke.TestNode')
28
29# connect the nodes.
30node_1.set_output(0, node_2.input(0))
31node_2.set_output(1, node_3.input(0))
32
33# auto layout the nodes.
34graph.auto_layout_nodes()
35
36# create a backdrop node and wrap it to node_1 and node_2.
37backdrop = graph.create_node('Backdrop')
38backdrop.wrap_nodes([node_1, node_2])
39
40
41# create the wrapper widget.
42class CustomNodeGraph(QtWidgets.QWidget):
43
44 def __init__(self, parent=None):
45 super(CustomNodeGraph, self).__init__(parent)
46 layout = QtWidgets.QVBoxLayout(self)
47 layout.setContentsMargins(0, 0, 0, 0)
48 layout.addWidget(graph.widget)
49
50 @staticmethod
51 def _set_nuke_zero_margin(widget_object):
52 """
53 Foundry Nuke hack for "nukescripts.panels.registerWidgetAsPanel" to
54 remove the widget contents margin.
55
56 sourced from: https://gist.github.com/maty974/4739917
57
58 Args:
59 widget_object (QtWidgets.QWidget): widget object.
60 """
61 if widget_object:
62 parent_widget = widget_object.parentWidget().parentWidget()
63 target_widgets = set()
64 target_widgets.add(parent_widget)
65 target_widgets.add(parent_widget.parentWidget().parentWidget())
66 for widget_layout in target_widgets:
67 widget_layout.layout().setContentsMargins(0, 0, 0, 0)
68
69 def event(self, event):
70 if event.type() == QtCore.QEvent.Type.Show:
71 try:
72 self._set_nuke_zero_margin(self)
73 except Exception:
74 pass
75 return super(CustomNodeGraph, self).event(event)
76
77# register the wrapper widget as a panel in Nuke.
78panels.registerWidgetAsPanel(
79 widget='CustomNodeGraph',
80 name='Custom Node Graph',
81 id='nodegraphqt.graph.CustomNodeGraph'
82)